Variables
Containers that store data.
Variables in Python are like containers that store data. They have a name, and they hold values that can be changed or used throughout your program. You can think of them as labeled boxes where you put different kinds of stuff, like numbers, text, or lists.
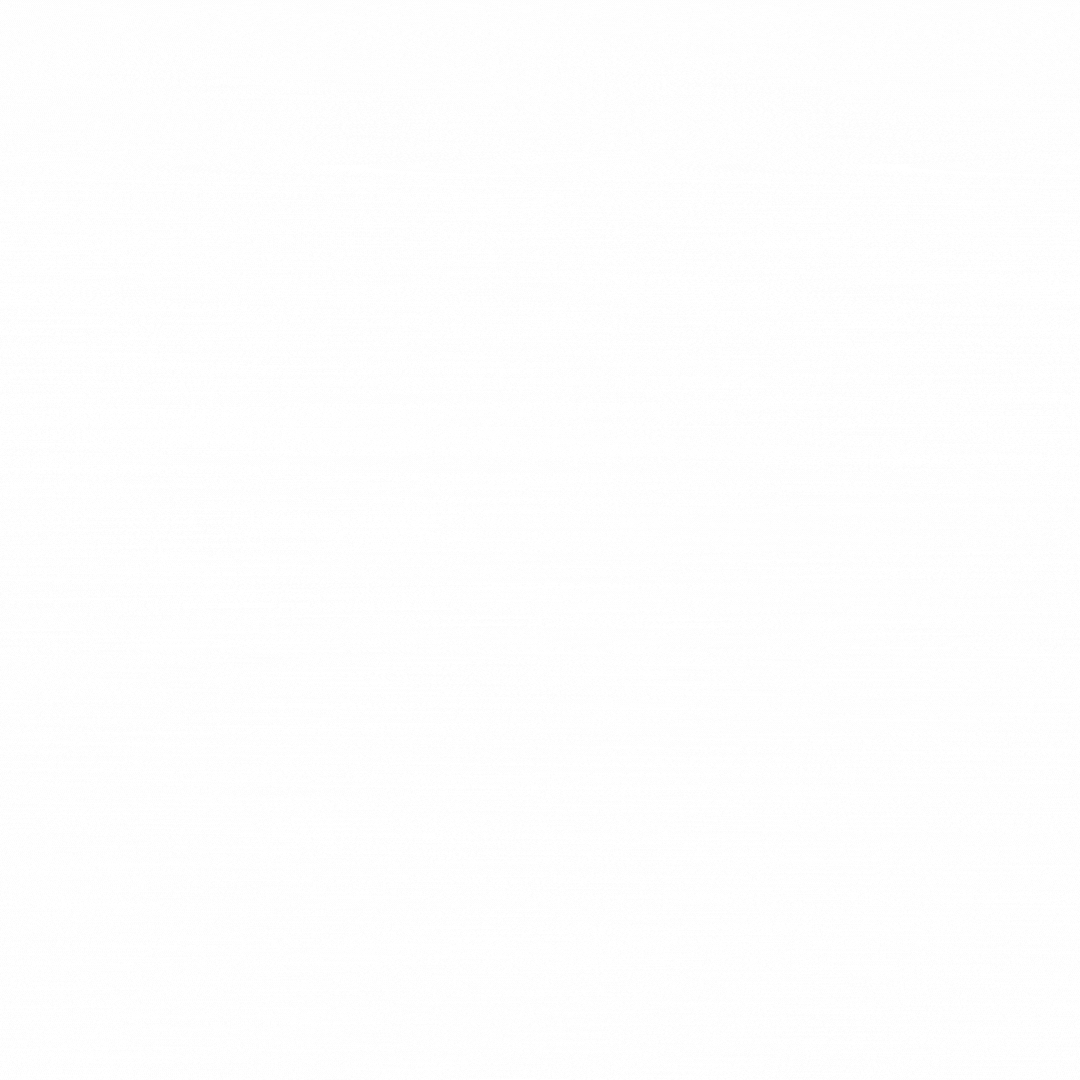
Key Features of Python Variables:
- Dynamic Typing
- Python variables don’t need a type declaration.
- The type is determined automatically when you assign a value.
Example:
x = 5 # x is an integer
name = "Jasmeet" # name is a string
pi = 3.14 # pi is a float
- Case Sensitivity
- Variable names are case-sensitive.
Age
andage
are two different variables.
Example:
age = 25
Age = 30
print(age) # Output: 25
print(Age) # Output: 30
- Reassignable
- You can assign a new value to the same variable.
Example:
x = 10
x = "Now a string"
print(x) # Output: Now a string
How to Create Variables in Python
-
Assignment
Use the=
operator to assign a value to a variable.
Example:age = 21 name = "John"
-
Multiple Assignments
Assign multiple variables in one line.
Example:a, b, c = 1, 2, 3 print(a, b, c) # Output: 1 2 3
Or assign the same value to multiple variables:
x = y = z = 10 print(x, y, z) # Output: 10 10 10
Rules for Naming Variables
- Variable names can contain letters, numbers, and underscores
_
, but must start with a letter or_
. - Cannot use Python keywords (like
if
,for
,print
, etc.). - Avoid starting with numbers.
Valid Names:my_variable = 10 _value = 20
Invalid Names:
1st_var = 10 # Starts with a number
if = 20 # Reserved keyword
Variables can hold different types of data, we will learn more about data types in the next chapter.
Good Practices for Variables
-
Use descriptive names:
Bad:x = 50
Good:total_items = 50
-
Follow snake_case naming convention:
Example:student_name
,average_score
-
Use constants (all caps) for fixed values:
Example:PI = 3.14