Types of Errors
Types of errors in Python
Errors are supposed to happen: input given not of set datatype, dividing by zero, and more. It is important to learn what is the error and how to avoid it for debugging. In Python, errors are broadly categorized into syntax errors and exceptions (runtime errors).
Below is a list of common error types:
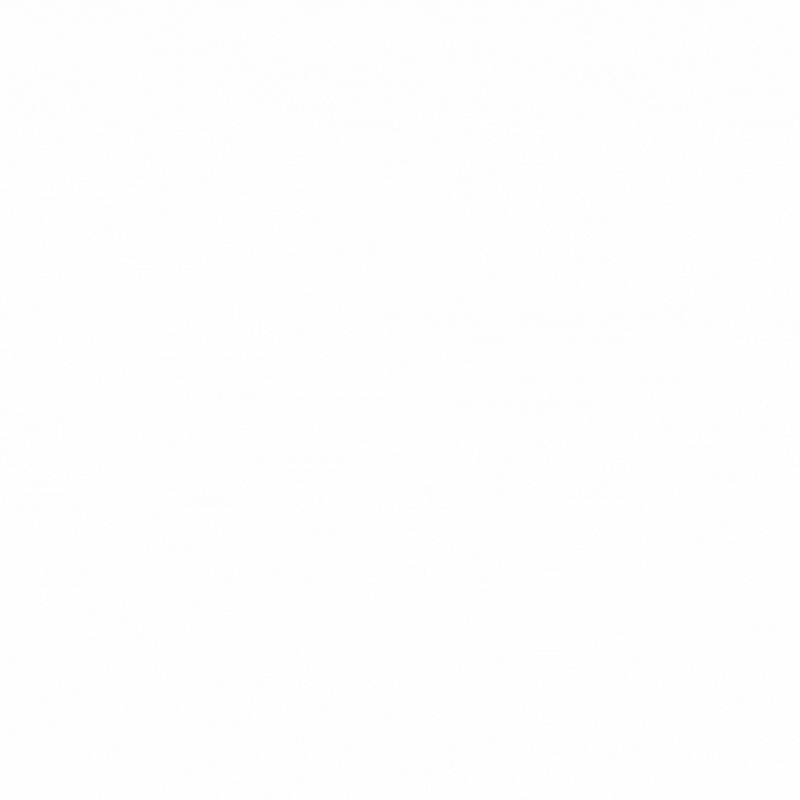
Syntax Errors
These occur when Python encounters incorrect syntax in the code.
print("Hello" # Missing closing parenthesis
Error: SyntaxError: unexpected EOF while parsing
Exceptions (Runtime Errors)
These occur while the program is running.
NameError
Occurs when trying to use a variable that hasn’t been defined.
print(my_variable) # my_variable is not defined
Error: NameError: name 'my_variable' is not defined
TypeError
Occurs when an operation is performed on an incompatible data type.
print("Hello" + 5) # String and integer cannot be concatenated
Error: TypeError: can only concatenate str (not "int") to str
ValueError
Occurs when a function receives an argument of the correct type but an inappropriate value.
int("hello") # Cannot convert a non-numeric string to an integer
Error: ValueError: invalid literal for int() with base 10: 'hello'
IndexError
Occurs when trying to access an index that does not exist in a list.
numbers = [1, 2, 3]
print(numbers[5]) # Index out of range
Error: IndexError: list index out of range
KeyError
Occurs when trying to access a non-existent key in a dictionary.
my_dict = {"name": "Jasmeet"}
print(my_dict["age"]) # "age" key does not exist
Error: KeyError: 'age'
AttributeError
Occurs when an invalid attribute is accessed for an object.
num = 10
num.append(5) # Integers do not have an 'append' method
Error: AttributeError: 'int' object has no attribute 'append'
ZeroDivisionError
Occurs when attempting to divide by zero.
print(10 / 0) # Division by zero is not allowed
Error: ZeroDivisionError: division by zero
FileNotFoundError
Occurs when attempting to open a file that does not exist.
with open("missing_file.txt", "r") as file:
content = file.read()
Error: FileNotFoundError: [Errno 2] No such file or directory: 'missing_file.txt'
ImportError / ModuleNotFoundError
Occurs when trying to import a module that doesn’t exist or isn’t installed.
import non_existent_module
Error: ModuleNotFoundError: No module named 'non_existent_module'
IndentationError
Occurs when indentation is incorrect in a Python script.
def my_function():
print("Hello") # Missing indentation
Error: IndentationError: expected an indented block
Other Common Exceptions
- MemoryError – When the system runs out of memory.
- OverflowError – When a numerical operation exceeds the limit.
- RuntimeError – When an operation fails for an unspecified reason.
- AssertionError – Raised when an
assert
statement fails.