Operators
Keywords to carry out special actions.
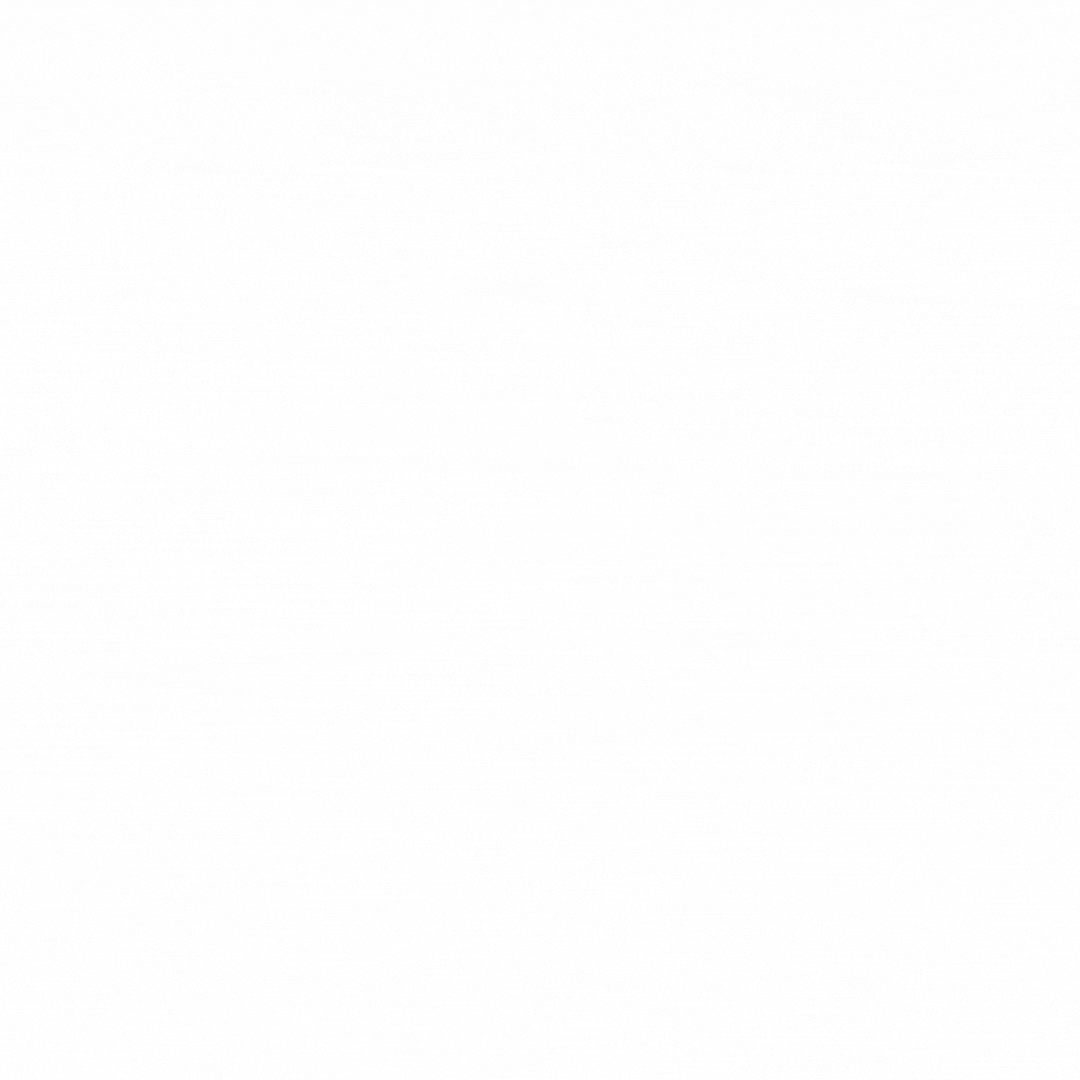
Python has a variety of operators that perform operations on variables and values. These operators are categorized based on their functionality:
Arithmetic Operators
Used to perform mathematical operations:
Operator | Description | Example |
---|---|---|
+ | Addition | a + b |
- | Subtraction | a - b |
* | Multiplication | a * b |
/ | Division | a / b |
% | Modulus (remainder) | a % b |
** | Exponentiation | a ** b (a^b) |
// | Floor Division | a // b |
a, b = 10, 3
print(a + b) # 13
print(a ** b) # 1000
Comparison (Relational) Operators
Used to compare two values and return a boolean result:
Operator | Description | Example |
---|---|---|
= = | Equal to | a = = b |
! = | Not equal to | a ! = b |
> | Greater than | a > b |
< | Less than | a < b |
>= | Greater than or equal to | a >= b |
<= | Less than or equal to | a <= b |
a, b = 10, 3
print(a > b) # True
Logical Operators
Used to combine conditional statements:
Operator | Description | Example |
---|---|---|
and | Logical AND | a > 5 and b < 10 |
or | Logical OR | a > 5 or b < 10 |
not | Logical NOT | not(a > 5) |
a, b = 10, 3
print(a > 5 and b < 5) # True
Assignment Operators
Used to assign values to variables:
Operator | Description | Example |
---|---|---|
= | Assign | a = 10 |
+= | Add and assign | a += 5 |
-= | Subtract and assign | a -= 5 |
*= | Multiply and assign | a *= 5 |
%= | Modulus and assign | a %= 5 |
Membership Operators
Used to check if a value is part of a sequence:
Operator | Description | Example |
---|---|---|
in | Returns True if a value exists | 'a' in 'apple' |
not in | Returns True if it does not | 'x' not in 'apple' |
fruits = ["apple", "banana"]
print("apple" in fruits) # True
Identity Operators
Used to compare the memory locations of two objects:
Operator | Description | Example |
---|---|---|
is | Returns True if objects are identical (same memory location) | a is b |
is not | Returns True if objects are not identical | a is not b |
No questions available.