Data Types
Types of data a variable can hold.
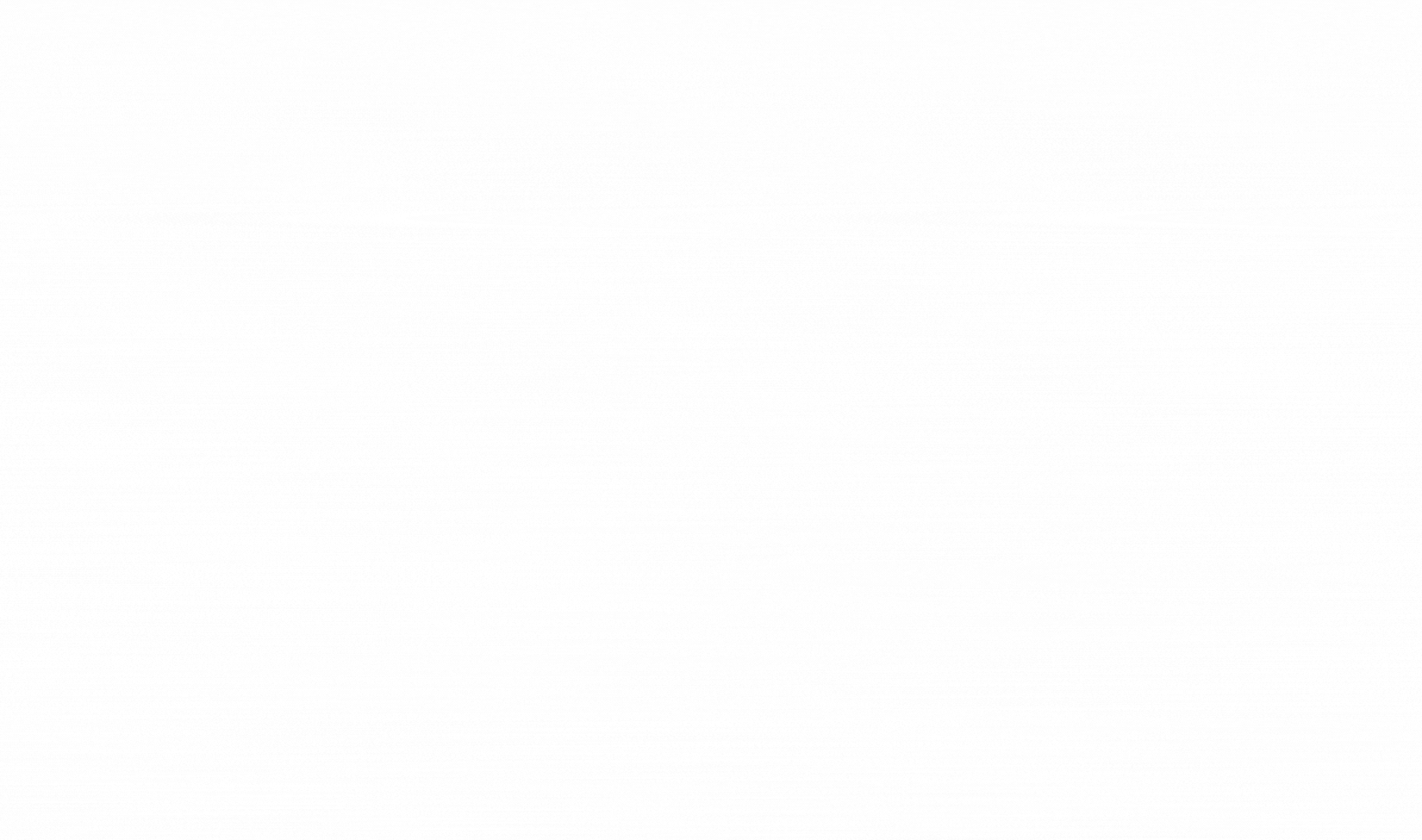
In Python, data types are classifications of data items that specify the type of value a variable can hold. Python's data types can be categorized into the following major groups:
Basic (or Built-in) Data Types
These are the fundamental data types used in Python:
- Numeric Types:
int
: Integer numbers (e.g.,1
,42
,-7
).float
: Floating-point numbers (e.g.,3.14
,-0.5
).complex
: Complex numbers (e.g.,3+4j
,2-3j
).
x = 10 # int
y = 3.14 # float
z = 1 + 2j # complex
- Text Type:
str
: Strings, or sequences of Unicode characters (e.g.,"Hello, World!"
).
name = "Jasmeet" # str
- Boolean Type:
bool
: Represents two values:True
orFalse
.
is_valid = True # bool
Sequence Types
These represent ordered collections of items:
- List (
list
): Mutable, ordered collection of items (e.g.,[1, 2, 3]
,["apple", "banana"]
). - Tuple (
tuple
): Immutable, ordered collection of items (e.g.,(1, 2, 3)
,("a", "b")
). - Range (
range
): Represents a sequence of numbers (e.g.,range(5)
produces0, 1, 2, 3, 4
).
In python, mutable objects can be changed after creation, while immutable objects cannot be changed after creation.
fruits = ["apple", "banana"] # list
coordinates = (10, 20) # tuple
Set Types
Unordered collections of unique items:
- Set (
set
): Mutable collection of unique items (e.g.,{1, 2, 3}
). - Frozenset (
frozenset
): Immutable version of a set.
unique_items = {1, 2, 3} # set
Mapping Type
Associations between keys and values:
- Dictionary (
dict
): Mutable collection of key-value pairs (e.g.,{"name": "Jasmeet", "age": 30}
).
person = {"name": "Jasmeet", "age": 30} # dict
Binary Types
Used for binary data manipulation:
- Bytes (
bytes
): Immutable sequences of bytes (e.g.,b"hello"
). - Bytearray (
bytearray
): Mutable sequences of bytes. - Memoryview (
memoryview
): Views memory of another binary object without copying.
Specialized Data Types (via Libraries)
Python also supports additional data types through libraries, such as:
datetime
: For handling date and time.Decimal
andFraction
: For precise arithmetic.
Each data type in Python has its own methods and operations that make it versatile and easy to use.
No questions available.