Control Flow
The order in which code is executed in python
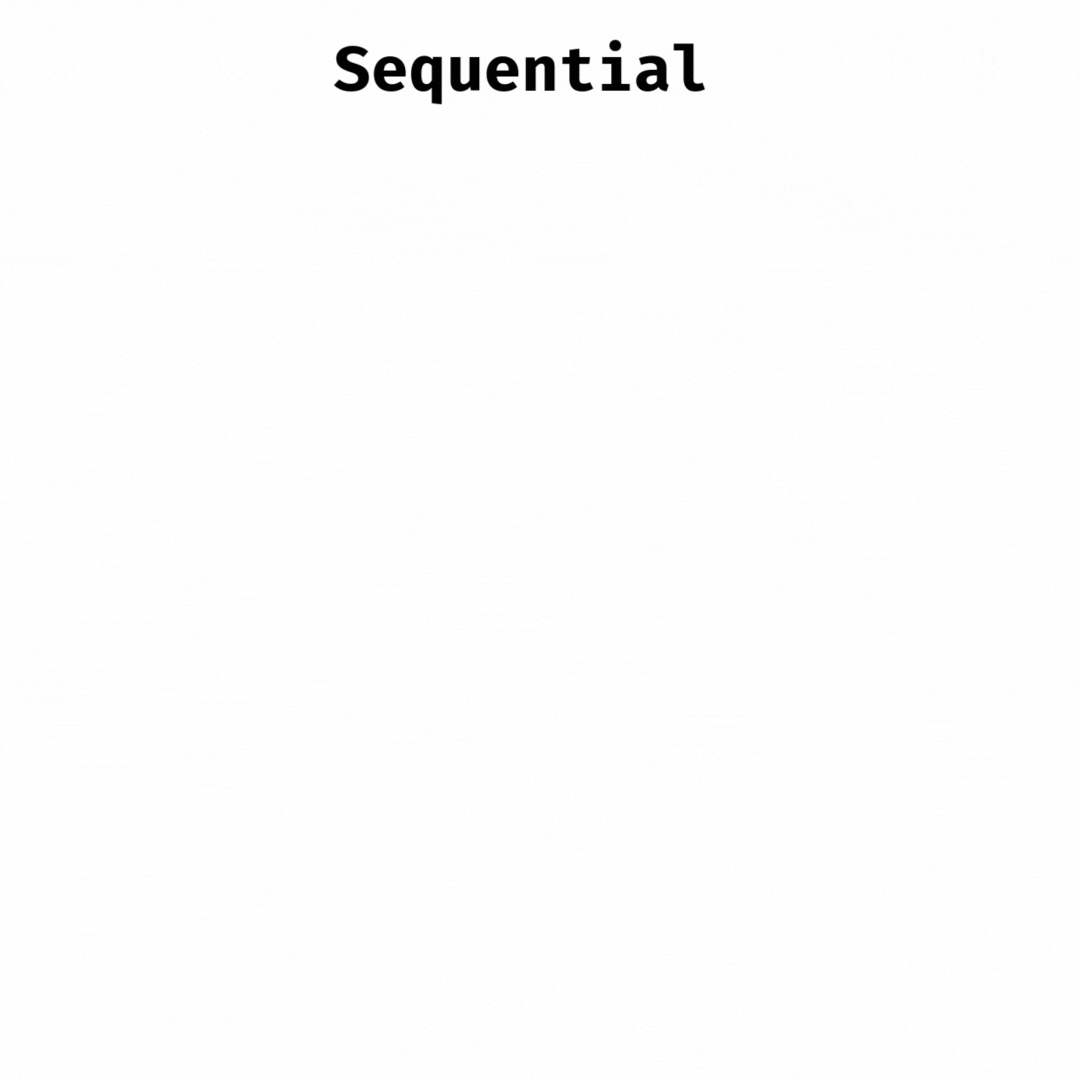
Control flow in Python refers to the mechanisms that allow you to dictate the order in which code is executed based on conditions or loops.
Python's control flow constructs include conditional statements, loops, and control statements like break
, continue
, and pass
.
Here's a breakdown of these concepts:
Conditional Statements
Conditional statements allow the program to execute different blocks of code based on conditions.
if
Statement:x = 10 if x > 5: print("x is greater than 5")
Note: Block of code after if statement is indented.
-
if-else
Statement:x = 3 if x > 5: print("x is greater than 5") else: print("x is not greater than 5")
-
if-elif-else
Statement:x = 7 if x > 10: print("x is greater than 10") elif x == 7: print("x is exactly 7") else: print("x is less than 10 but not 7")
Loops
Loops are used to execute a block of code repeatedly.
-
for
Loop: Iterates over a sequence.for i in range(5): print(i) # Outputs 0, 1, 2, 3, 4
-
while
Loop: Executes as long as a condition isTrue
.count = 0 while count < 5: print(count) count += 1
Control Statements
These statements alter the normal flow of a loop.
-
break
: Exits the loop prematurely.for i in range(10): if i == 5: break print(i) # Outputs 0, 1, 2, 3, 4
-
continue
: Skips the rest of the code in the current iteration and moves to the next iteration.for i in range(5): if i == 2: continue print(i) # Outputs 0, 1, 3, 4
-
pass
: Does nothing; acts as a placeholder.for i in range(5): if i == 2: pass print(i) # Outputs 0, 1, 2, 3, 4
##try-except
for Exception Handling
While not a traditional control flow mechanism, exception handling allows you to handle errors gracefully.
x= a
try:
num = int(x)
print(num)
except:
print("That's not a number!") # Output : That's not a number!
we will learn more about try and except in error handling section.
Nested Control Flow
You can nest if
, loops, and control statements within one another to create complex logic.
for i in range(3):
for j in range(2):
if i == j:
print(f"i equals j at {i}")