Error Handling
Handling errors in Python using try and except
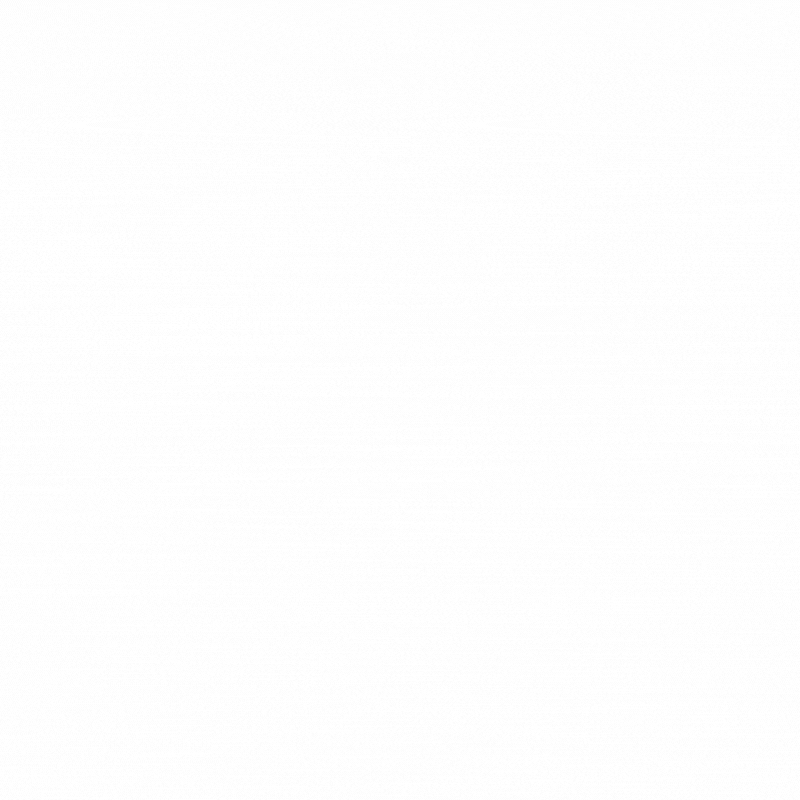
There are scenarios where you need to include code that might throw errors but cannot avoid it because it is critical to the program. That is where error handling comes to picture.
Error handling in Python involves using try, except, else, and finally blocks to manage exceptions effectively and ensure the program continues to run smoothly. Here's a breakdown of how error handling works:
Basic Syntax
try:
# Code that may raise an exception
risky_operation()
except SomeError as e:
# Handle the exception
print(f"An error occurred: {e}")
Common Exception Handling Blocks
try
: The block of code you want to execute.except
: The block that runs if an exception occurs.else
: (Optional) Runs if no exceptions are raised.finally
: (Optional) Always runs, regardless of exceptions.
Example with All Blocks
try:
num = int(input("Enter a number: "))
print(10 / num)
except ValueError:
print("Please enter a valid integer.")
except ZeroDivisionError:
print("Division by zero is not allowed.")
else:
print("The operation was successful.")
finally:
print("Execution complete.")
Catching Multiple Exceptions
You can catch multiple exceptions in one except
block:
try:
file = open("nonexistent.txt", "r")
content = file.read()
except (FileNotFoundError, IOError) as e:
print(f"An error occurred: {e}")
raise
to Re-raise Exceptions
Using You can raise exceptions deliberately or propagate them after handling:
try:
raise ValueError("Custom error message")
except ValueError as e:
print(e)
raise # Re-raise the exception
Defining Custom Exceptions
You can define custom exceptions by subclassing the Exception
class:
class CustomError(Exception):
pass
try:
raise CustomError("This is a custom error.")
except CustomError as e:
print(e)
Best Practices
- Always catch specific exceptions rather than a generic
Exception
unless absolutely necessary. - Use
finally
for cleanup tasks, such as closing files or releasing resources. - Log errors for better debugging and monitoring in production systems.
No questions available.