Functions
Predefined and reusable code blocks in Python
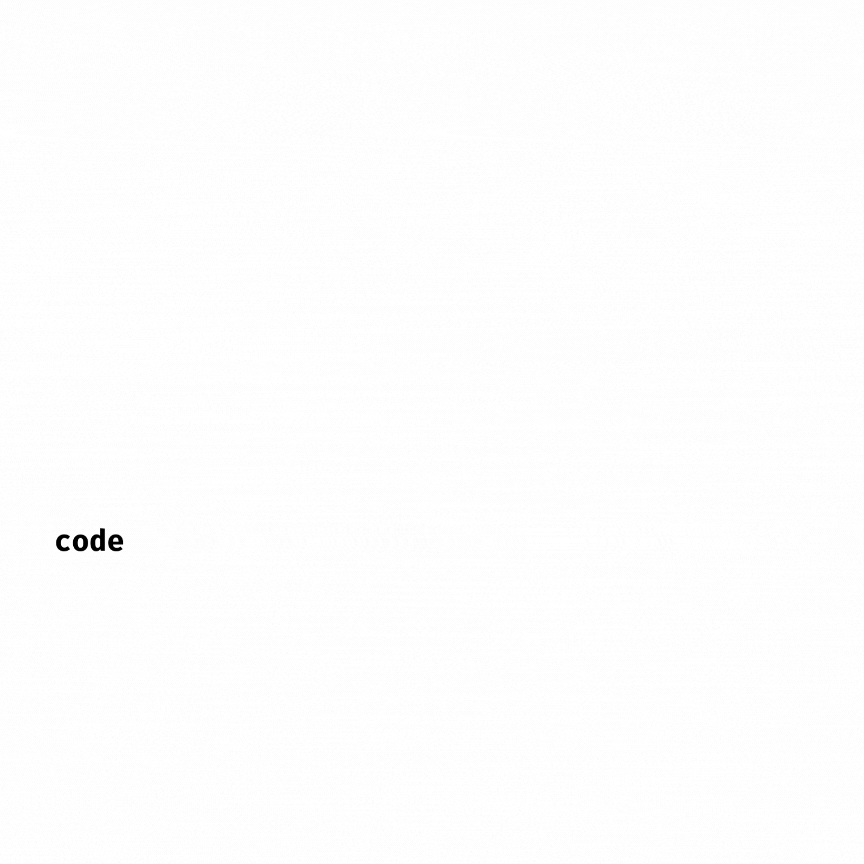
Functions in Python are blocks of reusable code that perform a specific task. They help in organizing code, making it more modular, and improving readability. Functions are especially useful when the same task needs to be performed multiple times. Here's a breakdown of key aspects of functions in Python:
Defining a Function
Functions in Python are defined using the def
keyword.
def greet():
print("Hello, World!")
Calling a Function
To execute a function, use its name followed by parentheses.
greet() # Output: Hello, World!
Function Parameters
Functions can accept input in the form of parameters, which allow them to process dynamic data.
def greet(name):
print(f"Hello, {name}!")
greet("Jasmeet") # Output: Hello, Jasmeet!
Default Parameters You can define default values for parameters, making them optional when calling the function.
def greet(name="Guest"):
print(f"Hello, {name}!")
greet() # Output: Hello, Guest!
greet("Chris") # Output: Hello, Chris!
Return Statement
Functions can return a value using the return
keyword.
def add(a, b):
return a + b
result = add(5, 3)
print(result) # Output: 8
Variable Scope
Variables defined inside a function have local scope and are not accessible outside the function.
y = 11 # This is a Global variable
def func():
x = 10 # Local variable
print(y)
func() # Output : 11
# print(x) # This will raise an error since x is not defined outside the function.
Lambda Functions
Lambda functions are anonymous, single-expression functions. They're defined using the lambda
keyword.
add = lambda x, y: x + y
print(add(5, 3)) # Output: 8
Nested Functions
Functions can be defined inside other functions.
def outer_function():
def inner_function():
print("Inner function")
inner_function()
outer_function()
Higher-Order Functions
Functions can accept other functions as arguments or return them.
def apply_function(func, value):
return func(value)
result = apply_function(lambda x: x**2, 5)
print(result) # Output: 25
Decorators
Decorators are special functions that modify the behavior of another function.
def decorator(func):
def wrapper():
print("Before the function call")
func()
print("After the function call")
return wrapper
@decorator
def say_hello():
print("Hello!")
say_hello()
Built-in Functions
Python provides several built-in functions, such as len()
, max()
, min()
, print()
, sum()
, etc.
numbers = [1, 2, 3, 4, 5]
print(sum(numbers)) # Output: 15
Functions are a cornerstone of Python programming, allowing you to build efficient, maintainable, and scalable code.