Basic Syntax
let's get started with syntax in Python
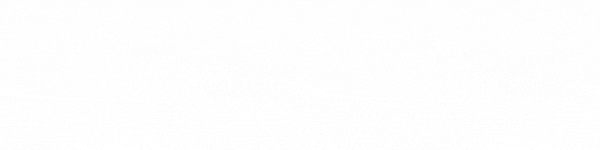
Python syntax is the set of rules that define how Python programs are written and understood by the Python interpreter. It’s designed to be simple, readable, and straightforward.
Here’s an overview of the key components of Python syntax:
Whitespace and Indentation
- Indentation is crucial in Python. Unlike many other languages, Python uses indentation (spaces or tabs) to define blocks of code.
- No curly braces
{}
or semicolons;
are needed. Indentation keeps the code clean and readable.
Example:
if 5 > 3:
print("Five is greater than three") # This line is indented
Variables and Assignment
- Variables don’t need to be declared with a type. Python figures it out based on the value you assign.
- Assignment is done using the
=
operator.
Example:
x = 10 # Assigns 10 to the variable x
name = "Jasmeet" # Assigns a string to the variable name
Data Types
Python has built-in data types like:
- Numbers:
int
,float
- Strings:
str
- Lists:
list
- Tuples:
tuple
- Dictionaries:
dict
- Booleans:
bool
Example:
age = 25 # Integer
pi = 3.14 # Float
greeting = "Hello!" # String
colors = ["red", "blue"] # List
Comments
- Comments are notes for developers and ignored by Python. They start with
#
. - Multi-line comments use triple quotes
'''
or"""
.
Example:
# This is a single-line comment
"""
This is a
multi-line comment
"""
Input and Output
- Use
input()
to take input from the user. - Use
print()
to display output.
Example:
name = input("What is your name? ")
print(f"Hello, {name}!")
Importing Libraries
You can use libraries to extend Python’s functionality with the import
keyword.
Example:
import math
print(math.sqrt(16)) # Output: 4.0
No questions available.