Clases
A blueprint for creating objects in Python.
Introduction
In Python, a class is a blueprint for creating objects, which are instances of the class. A class can include attributes (variables) and methods (functions) that define the behavior and properties of the objects.
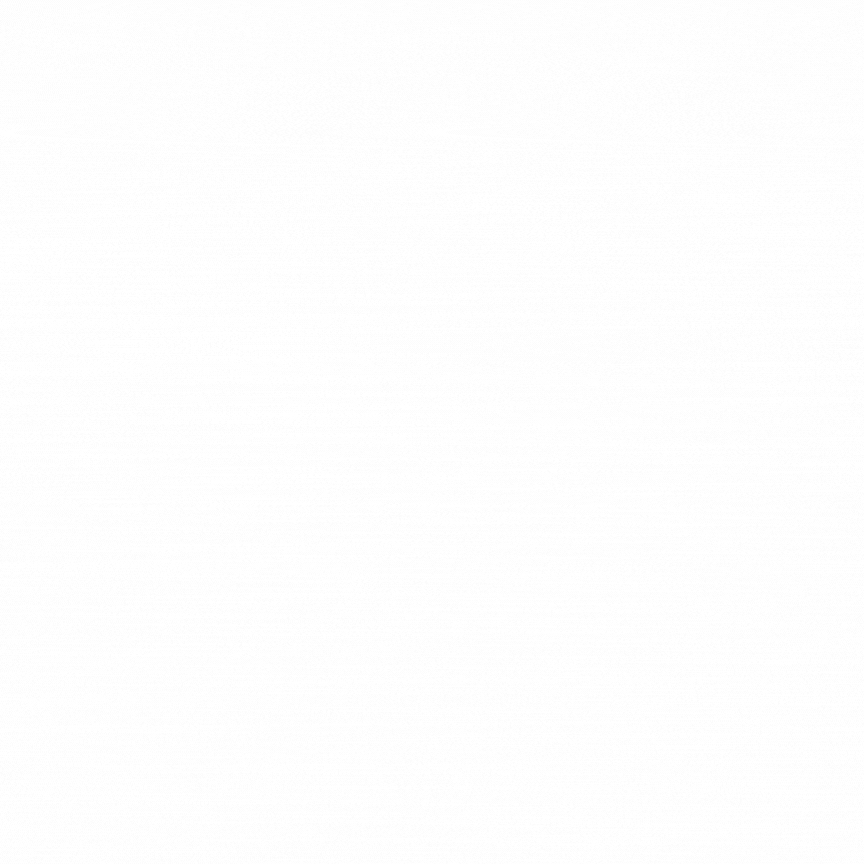
Imagine you're building something with Lego bricks. Each brick is like a small, reusable piece that can be used to create something larger, like a house, car, or spaceship. In python, a class in Python is like a blueprint for making these Lego pieces.
Here’s how it works:
-
Blueprint for Objects: A class is a recipe or template that tells Python how to create something. For example, if you want to create multiple cars in your program, you can define a "Car" class as the blueprint.
-
Objects from Classes: Once you have a class, you can create objects from it. These objects are like the actual cars made from the blueprint. Each object can have its own details, like color or brand, but all follow the same blueprint.
-
What Classes Contain: A class typically contains:
- Attributes (Data): These are the properties of the objects, like the color or speed of a car.
- Methods (Actions): These are the things the object can do, like start, stop, or honk.
Example: A Class in Action
Here’s a simple example in Python:
# Blueprint (Class)
class Car:
def __init__(self, brand, color):
self.brand = brand # Attribute: brand
self.color = color # Attribute: color
def start(self): # Method: start
print(f"The {self.color} {self.brand} car is starting!")
# Making objects (Lego pieces)
car1 = Car("Toyota", "red") # A red Toyota
car2 = Car("Ford", "blue") # A blue Ford
# Using the objects
car1.start() # Output: The red Toyota car is starting!
car2.start() # Output: The blue Ford car is starting!
Let's break it down step by step:
Class Definition:
class Car:
Car
is the class name, acting as the blueprint for creating car objects.
Constructor (__init__
Method):
def __init__(self, brand, color):
self.brand = brand # Attribute: brand
self.color = color # Attribute: color
- The
__init__
method is called automatically when a new object is created from the class. brand
andcolor
are parameters used to initialize the attributesself.brand
andself.color
for each car object.
Method (start
):
def start(self):
print(f"The {self.color} {self.brand} car is starting!")
- The
start
method defines a behavior (action) for the car objects. It uses the attributesself.color
andself.brand
to print a message about the car starting.
Creating Objects:
car1 = Car("Toyota", "red") # A red Toyota
car2 = Car("Ford", "blue") # A blue Ford
car1
andcar2
are instances of theCar
class.- Each object is initialized with specific values for
brand
andcolor
.
Calling Methods:
car1.start() # Output: The red Toyota car is starting!
car2.start() # Output: The blue Ford car is starting!
- The
start
method is called on each object. - The
self
keyword in the method allows it to access the unique attributes (brand
andcolor
) of each object.
This showcases how you can use classes to create and manage objects with their own attributes and methods.
Key Concepts in Classes
- Attributes: Variables that belong to a class or an instance.
- Class attributes: Shared by all instances of the class.
- Instance attributes: Unique to each instance.
- Methods: Functions defined inside a class. They typically operate on the attributes of the object.
- Instance methods: Use self to access instance attributes and methods.
- Class methods: Use @classmethod and cls to modify class-level data.
- Static methods: Use @staticmethod and don't depend on self or cls.
-
Constructor (init): A special method called when an object is created.
-
Inheritance: Allows a class to inherit properties and methods from another class.
-
Encapsulation: Restricts access to certain parts of an object to enforce a controlled interface.
-
Polymorphism: Allows methods to have different behaviors in derived classes.
Why Use Classes?
- Reusability: Once you have a class, you can create as many objects as you want without rewriting code.
- Organization: Classes group related data and actions together, making programs easier to manage.
- Real-world Modeling: Classes help mimic real-world things, making programs more intuitive to design and understand.
In short, a class is like a master plan, and objects are the real-world things you create from it.