Spatial Data in SQL
Handling Spatial data.
Introduction
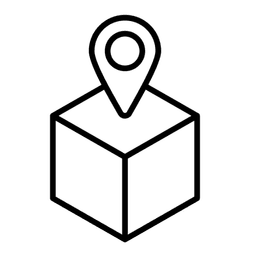
Spatial data refers to data that represents objects or features defined in a geographic space. In MS SQL, spatial data types are used to store and manipulate geographic and geometric data. These include coordinates, points, lines, and polygons representing locations, routes, or regions.
There are two primary spatial data types in MS SQL:
- Geometry: For flat, Euclidean space (e.g., a map projection).
- Geography: For curved, Earth-like space (based on the Earth’s shape).
This section will explore the use of spatial data in customer management scenarios, such as tracking customer locations, delivery routes, and service area coverage.
Spatial Data Types
MS SQL supports two main spatial data types:
GEOMETRY
: Used for 2D flat-plane data such as maps or engineering designs.GEOGRAPHY
: Stores geospatial data based on the Earth’s curvature, commonly used for geographic locations (latitude and longitude).
Both types allow you to define various shapes:
- Points: Single geographic locations.
- Linestrings: Sequences of points forming lines (e.g., roads).
- Polygons: Closed shapes representing areas (e.g., a city boundary).
Example: Defining customer locations as points.
CREATE TABLE CustomerLocations (
CustomerID INT PRIMARY KEY,
Name NVARCHAR(100),
Location GEOGRAPHY
);
Inserting and Querying Spatial Data
Spatial data is typically stored using well-known text (WKT) or well-known binary (WKB) representations. For example, points are defined by their latitude and longitude.
Example: Insert customer location data as a point.
INSERT INTO CustomerLocations (CustomerID, Name, Location)
VALUES (1, 'John Doe', GEOGRAPHY::Point(37.7749, -122.4194, 4326)); -- San Francisco, CA
In this example, the Point
function creates a geographic point with the latitude and longitude coordinates of San Francisco.
To retrieve and query spatial data:
SELECT Name, Location.ToString() AS Location
FROM CustomerLocations;
Spatial Functions
MS SQL provides several functions to analyze and work with spatial data. Some common functions include:
STDistance()
: Calculates the shortest distance between two geography points.STIntersection()
: Determines the intersection of two geometries or geographies.STArea()
: Calculates the area of a polygon.
Example: Calculate the distance between two customer locations.
DECLARE @Location1 GEOGRAPHY = GEOGRAPHY::Point(40.7128, -74.0060, 4326); -- New York City
DECLARE @Location2 GEOGRAPHY = GEOGRAPHY::Point(34.0522, -118.2437, 4326); -- Los Angeles
SELECT @Location1.STDistance(@Location2) AS DistanceInMeters;
This query calculates the straight-line distance (in meters) between New York and Los Angeles.
Spatial Indexing
Spatial indexes optimize queries that involve spatial data by allowing fast searching and filtering based on locations. Without indexing, spatial queries (e.g., finding nearby locations) can become slow as data size grows.
Example: Create a spatial index on the Location
column in the CustomerLocations
table.
CREATE SPATIAL INDEX SIndx_CustomerLocation
ON CustomerLocations(Location);
After creating the index, queries such as distance calculations or point-in-area searches will be much faster.
Common Use Cases
- Find the Nearest Store to a Customer Spatial queries can be used to find the closest store to a customer’s location.
Example: Find the closest store to a specific customer.
SELECT TOP 1 StoreName, StoreLocation.ToString() AS Location,
CustomerLocation.Location.STDistance(StoreLocation) AS Distance
FROM Stores
JOIN CustomerLocations AS CustomerLocation
ON CustomerLocation.CustomerID = 1 -- The customer to find a nearby store for
ORDER BY CustomerLocation.Location.STDistance(StoreLocation);
- Service Area Coverage In customer management, it's often necessary to determine if a customer resides within a service area (represented as a polygon). Spatial functions can calculate whether a point (customer location) falls inside a defined area.
Example: Check if a customer is inside a service area polygon.
DECLARE @ServiceArea GEOGRAPHY = GEOGRAPHY::STPolyFromText('POLYGON((...))', 4326); -- Define polygon
SELECT Name
FROM CustomerLocations
WHERE @ServiceArea.STContains(Location) = 1;
The STContains()
function checks whether a customer’s location is within the service area.
Spatial Data Visualizations
Spatial data can be exported to external tools like Power BI or integrated into map services for visualization. This allows businesses to visualize customer distributions, store coverage, or delivery routes directly on a map.
Example: Export customer locations to GeoJSON for map visualization.
SELECT Name, Location.STAsText() AS GeoData
FROM CustomerLocations;
This query exports spatial data in a format that can be visualized on tools like Google Maps or Power BI.
Conclusion
Spatial data in MS SQL opens the door to advanced geographic and geometric analysis.
By utilizing spatial data types like GEOMETRY
and GEOGRAPHY
, combined with spatial functions and indexing,
businesses can efficiently manage and analyze location-based information. From finding the nearest store to visualizing
customer distributions, spatial data offers powerful solutions for customer management scenarios.