List, Tuples, and Sets
Lists, tuples, and sets are fundamental data structures in Python
Lists, tuples, and sets are fundamental data structures in Python, each with unique properties and use cases. Let’s break them down with explanations, examples, and key differences.
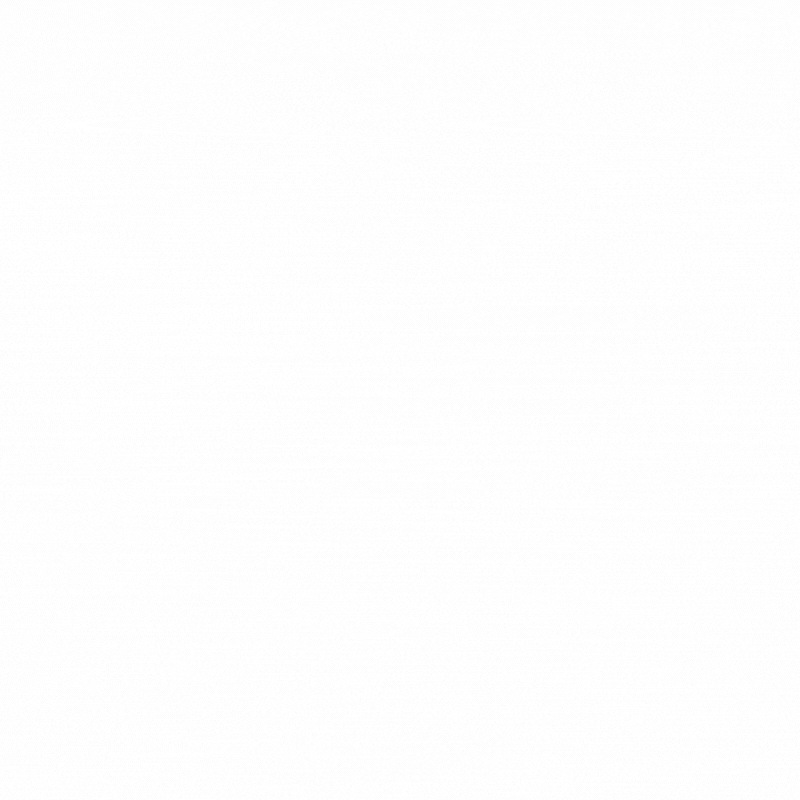
List
A list is an ordered, mutable (changeable) collection that allows duplicate values.
Key Features:
- Ordered (items maintain their sequence)
- Mutable (elements can be modified)
- Allows duplicate values
- Supports indexing and slicing
# Creating a list
fruits = ["apple", "banana", "cherry", "apple"]
print(fruits) # ['apple', 'banana', 'cherry', 'apple']
# Accessing elements
print(fruits[1]) # 'banana'
# Modifying elements
fruits[1] = "orange"
print(fruits) # ['apple', 'orange', 'cherry', 'apple']
# Adding elements
fruits.append("grape") # Adds at the end
fruits.insert(1, "mango") # Adds at index 1
print(fruits) # ['apple', 'mango', 'orange', 'cherry', 'apple', 'grape']
# Removing elements
fruits.remove("apple") # Removes first occurrence
print(fruits) # ['mango', 'orange', 'cherry', 'apple', 'grape']
# Iterating over a list
for fruit in fruits:
print(fruit)
Tuple
A tuple is an ordered, immutable (unchangeable) collection that allows duplicate values.
Key Features:
- Ordered (items maintain their sequence)
- Immutable (cannot modify elements after creation)
- Allows duplicate values
- Supports indexing and slicing
# Creating a tuple
numbers = (1, 2, 3, 4, 2)
print(numbers) # (1, 2, 3, 4, 2)
# Accessing elements
print(numbers[0]) # 1
# Tuples are immutable, so modification is not allowed
# numbers[0] = 10 # TypeError: 'tuple' object does not support item assignment
# However, we can concatenate tuples
new_numbers = numbers + (5, 6)
print(new_numbers) # (1, 2, 3, 4, 2, 5, 6)
# Tuple unpacking
a, b, c, d, e = numbers
print(a, b, c, d, e) # 1 2 3 4 2
Sets
A set is an unordered, mutable collection of unique elements.
Key Features:
- Unordered (items do not maintain sequence)
- Mutable (can add/remove elements)
- Does not allow duplicate values
- Does not support indexing or slicing
# Creating a set
my_set = {1, 2, 3, 4, 2, 3} # Duplicates are automatically removed
print(my_set) # {1, 2, 3, 4}
# Adding elements
my_set.add(5)
print(my_set) # {1, 2, 3, 4, 5}
# Removing elements
my_set.remove(2)
print(my_set) # {1, 3, 4, 5}
# Checking membership
print(3 in my_set) # True
print(6 in my_set) # False
# Set operations
A = {1, 2, 3}
B = {3, 4, 5}
print(A.union(B)) # {1, 2, 3, 4, 5}
print(A.intersection(B)) # {3}
print(A.difference(B)) # {1, 2}
Key Differences Between List, Tuple, and Set
Feature | List | Tuple | Set |
---|---|---|---|
Order | Ordered | Ordered | Unordered |
Mutability | Mutable (changeable) | Immutable (unchangeable) | Mutable (can add/remove) |
Duplicates | Allowed | Allowed | Not allowed |
Indexing | Yes | Yes | No |
Use Case | When modification is needed | When data must stay fixed | When unique values are required |
When to Use What?
✅ Use a list when you need an ordered collection with the ability to modify elements.
✅ Use a tuple when you need an ordered collection that should remain unchanged.
✅ Use a set when you need a collection of unique elements and don't care about order.