Dictionaries
Collection of key-value pairs in Python
A dictionary in Python is a collection of key-value pairs where:
- Each key is unique: Dictionaries cannot have two items with the same key.
- Keys are immutable (strings, numbers, or tuples). That mean keys can not be changed.
- Values can be any data type (list, tuple, another dictionary, etc.).
Dictionaries are unordered in Python versions before 3.7, but from Python 3.7+, they maintain insertion order.
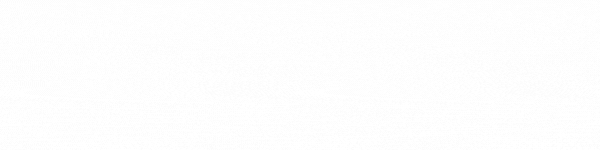
Creating a Dictionary
# Using curly braces {}
student = {"name": "Jasmeet", "age": 25, "city": "New York"}
# Using the dict() function
student2 = dict(name="Chris", age=30, city="London")
print(student)
print(student2)
Output:
{'name': 'Jasmeet', 'age': 25, 'city': 'New York'}
{'name': 'Chris', 'age': 30, 'city': 'London'}
Accessing Dictionary Values
dict["key"]
raises an error if the key doesn’t exist.dict.get("key")
returns None if the key doesn’t exist.
student = {"name": "Jasmeet", "age": 25, "city": "New York"}
print(student["name"]) # Jasmeet
print(student.get("age")) # 25
Adding & Updating Dictionary Values
student["age"] = 26 # Update existing value
student["gender"] = "Female" # Add new key-value pair
print(student)
Output:
{'name': 'Jasmeet', 'age': 26, 'city': 'New York', 'gender': 'Female'}
Removing Elements
student.pop("city") # Removes 'city'
del student["age"] # Deletes 'age'
student.clear() # Removes all elements
print(student)
Looping Through a Dictionary
student = {"name": "Jasmeet", "age": 25, "city": "New York"}
# Loop through keys
for key in student:
print(key, "->", student[key])
# Loop through values
for value in student.values():
print(value)
# Loop through key-value pairs
for key, value in student.items():
print(key, ":", value)
Dictionary Methods
You can use built-in Python methods on dictionaries.
Method | Description | Example |
---|---|---|
dict.get(key, default) | Returns value for key, else default | student.get("age", 20) |
dict.keys() | Returns all keys | student.keys() |
dict.values() | Returns all values | student.values() |
dict.items() | Returns key-value pairs | student.items() |
dict.pop(key) | Removes a key and returns value | student.pop("age") |
dict.update(new_dict) | Merges another dictionary | student.update({"gender": "Female"}) |
Nested Dictionary
A dictionary having another dictionaries as a values.
students = {
"Jasmeet": {"age": 25, "city": "New York"},
"Chris": {"age": 30, "city": "London"}
}
print(students["Jasmeet"]["city"]) # New York
Dictionary Comprehension
Dictionary Comprehension is a concise way to create dictionaries in Python using a single line of code.
# using for loop
squares = {x: x**2 for x in range(1, 6)}
print(squares)
# Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
# updating values
students = {"Jasmeet": 85, "Chris": 72, "Charlie": 90}
# Add 5 bonus points to each student
updated_scores = {name: score + 5 for name, score in students.items()}
print(updated_scores)
# Output: {'Jasmeet': 90, 'Chris': 77, 'Charlie': 95}
Why Use Dictionaries?
✅ Fast lookups
✅ Easy data manipulation
✅ Stores structured data efficiently
No questions available.