Ultimate guide to SQL loops.
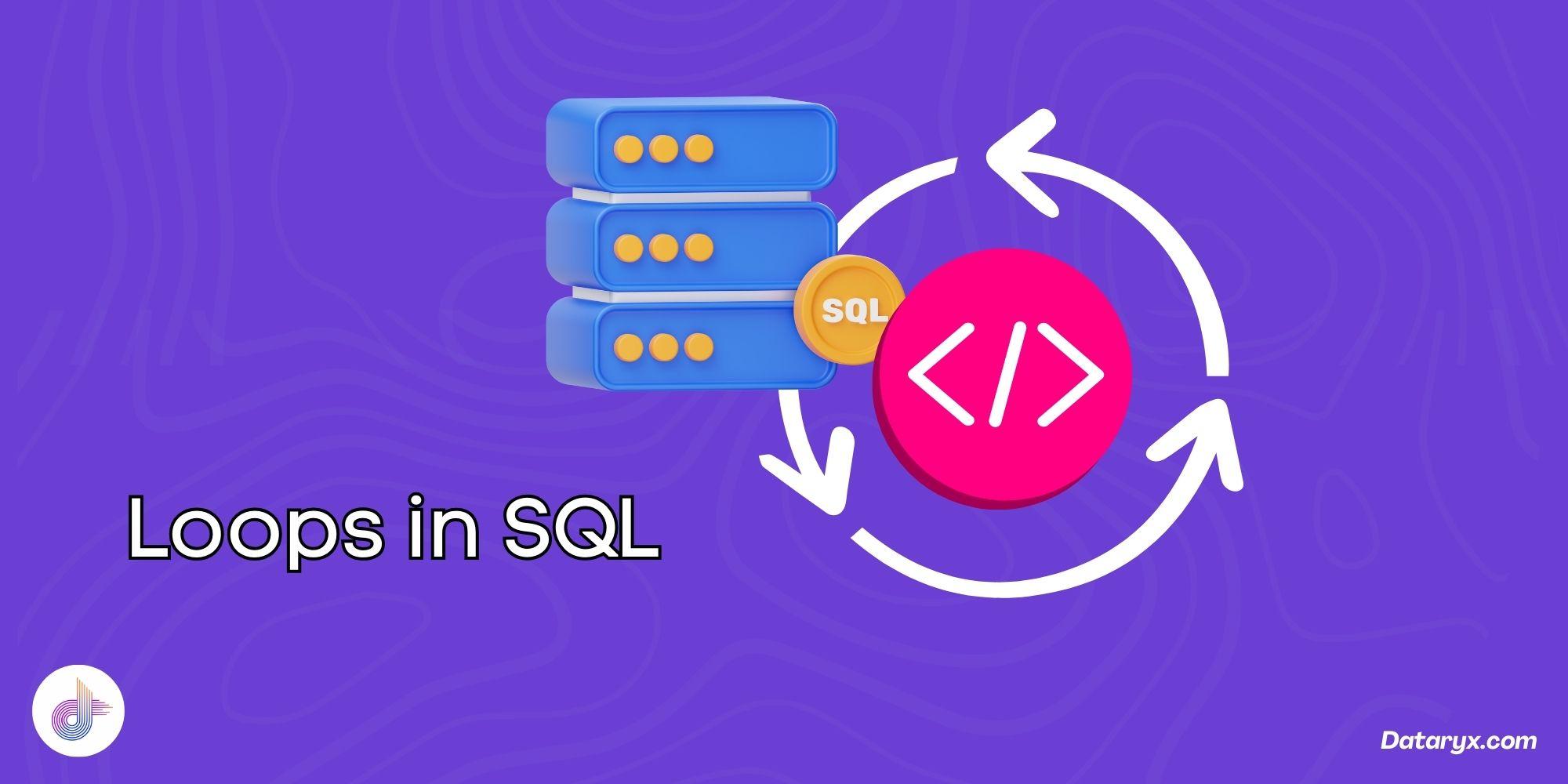
After learning the basics of SQL, it's essential to continue exploring advanced topics to enhance your query-writing skills and deepen your knowledge.
As a data professional, I often find myself using SQL loops frequently. They are incredibly helpful for automating repetitive tasks, processing large datasets, and managing complex data operations efficiently.
Here’s a complete guide to using loops in SQL that you can refer to for mastering them and using them proficiently.
What are SQL Loops?
SQL loops are structures that enable repetitive execution of a set of SQL statements until a specific condition is met.
They’re commonly used in T-SQL (SQL Server), PL/pgSQL (PostgreSQL), and PL/SQL (Oracle).
Types of Loops in SQL
a. WHILE Loop
Executes a block of code as long as a specified condition is TRUE
.
DECLARE @Counter INT = 1;
WHILE @Counter <= 5
BEGIN
PRINT 'Iteration ' + CAST(@Counter AS VARCHAR);
SET @Counter = @Counter + 1;
END;
Use Case: Iterating over a set of records or performing a repetitive task.
b. FOR Loop (PL/pgSQL and PL/SQL)
Executes a block of code for a predefined range or set of values.
FOR counter IN 1..5 LOOP
RAISE NOTICE 'Iteration %', counter;
END LOOP;
Use Case: Running through a fixed range of numbers or records.
c. Cursor FOR Loop (PL/pgSQL and PL/SQL)
Iterates through each row in a result set using a cursor.
FOR record IN SELECT * FROM employees LOOP
RAISE NOTICE 'Employee ID: %', record.emp_id;
END LOOP;
Use Case: Processing each row in a query result.
d. LOOP with EXIT Condition (PL/pgSQL and PL/SQL)
A generic loop structure that relies on an explicit exit condition.
LOOP
EXIT WHEN counter > 5;
RAISE NOTICE 'Iteration %', counter;
counter := counter + 1;
END LOOP;
Use Case: Flexible iteration when conditions are complex.
Key Components of SQL Loops
- Control Variables: Variables used to track iterations (e.g.,
@Counter
). - Conditions: Boolean expressions controlling the loop.
- Exit Mechanisms: Ways to terminate the loop, such as
EXIT
,BREAK
, orCONTINUE
.
Advanced Tips and Best Practices
- Avoid Infinite Loops: Ensure your loop has a clear exit condition.
- Optimize Performance: Use loops sparingly in SQL; operations like joins, CTEs, and set-based queries are usually more efficient.
- Error Handling: Include error-handling mechanisms like
TRY...CATCH
blocks to manage exceptions.
Common Use Cases for SQL Loops
- Batch Processing: Update or process records in smaller chunks to avoid locking or performance issues.
- Dynamic Query Execution: Build and execute dynamic SQL queries iteratively.
- Data Validation: Check and process rows in a result set conditionally.
- Complex Aggregations: Perform custom aggregations that can’t be achieved with built-in SQL functions.
SQL Loops in Different RDBMS
Feature | T-SQL (SQL Server) | PL/pgSQL (PostgreSQL) | PL/SQL (Oracle) |
---|---|---|---|
WHILE Loop | ✅ | ✅ | ✅ |
FOR Loop | ❌ | ✅ | ✅ |
Cursor FOR Loop | ✅ | ✅ | ✅ |
LOOP with EXIT | ❌ | ✅ | ✅ |
Limitations of SQL Loops
- Performance Bottlenecks: Loops are row-by-row operations (RBAR - Row By Agonizing Row) and can be slow for large datasets.
- Prefer Set-Based Logic: Where possible, use SQL joins, CTEs, and aggregate functions instead of loops.
Example: Batch Update with WHILE Loop
DECLARE @BatchSize INT = 100;
DECLARE @StartRow INT = 1;
WHILE @StartRow <= (SELECT COUNT(*) FROM large_table)
BEGIN
UPDATE TOP (@BatchSize) large_table
SET status = 'processed'
WHERE status = 'pending';
SET @StartRow = @StartRow + @BatchSize;
END;
Debugging and Logging in Loops
-
Use
PRINT
(T-SQL) orRAISE NOTICE
(PL/pgSQL) to log progress. -
Leverage temporary tables to store intermediate results for review.
Loops are powerful but should be used wisely. Whenever possible, prioritize set-based operations for efficiency.